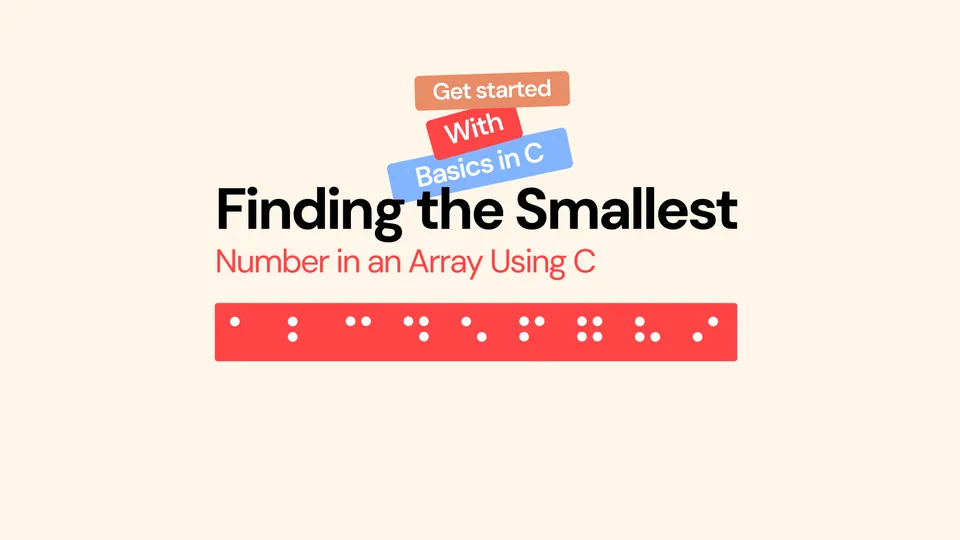
π Introduction
In this blog, weβll explore a simple yet essential algorithm in C programming: finding the smallest number in an array. This basic concept is crucial for understanding more complex algorithms and serves as a foundational step in mastering array manipulation. Weβll walk through the code, explain each part, and provide examples with outputs to solidify your understanding.
π Code Explanation
Hereβs a C program that allows the user to input an array and then finds the smallest number in that array.
#include<stdio.h>
int main() {
int i, n, s;
// Taking input for the length of the array
printf("Enter length of the array: ");
scanf("%d", &n);
int a[n]; // Declaring an array of size n
// Taking input for array elements
printf("Enter values into the array:\n");
for(i = 0; i < n; i++) {
printf("a[%d]: ", i);
scanf("%d", &a[i]);
}
s = a[0]; // Initializing the smallest number as the first element
// Loop to find the smallest number
for(i = 1; i < n; i++) {
if(s > a[i]) {
s = a[i]; // Updating the smallest number
}
}
// Printing the smallest number
printf("Smallest number in the array is %d\n", s);
return 0;
}
π Example and Output
Letβs take a closer look at how this program works with an example.
Input:
Enter length of the array: 5
Enter values into the array:
a[0]: 10
a[1]: 3
a[2]: 56
a[3]: 2
a[4]: 7
Output:
Smallest number in the array is 2
In this example, the program first asks the user to enter the number of elements in the array. Then, it prompts the user to input the values for each element. The program processes these values to find and print the smallest number, which is 2
in this case.
π Conclusion
Finding the smallest number in an array is a basic yet fundamental task in programming. It involves comparing elements and updating the minimum value as you progress through the array. Understanding this concept is crucial for more advanced topics such as sorting and searching algorithms. Try experimenting with different array sizes and values to see how the program performs in various scenarios.
Feel free to share your thoughts or ask questions in the comments below. Happy coding! π