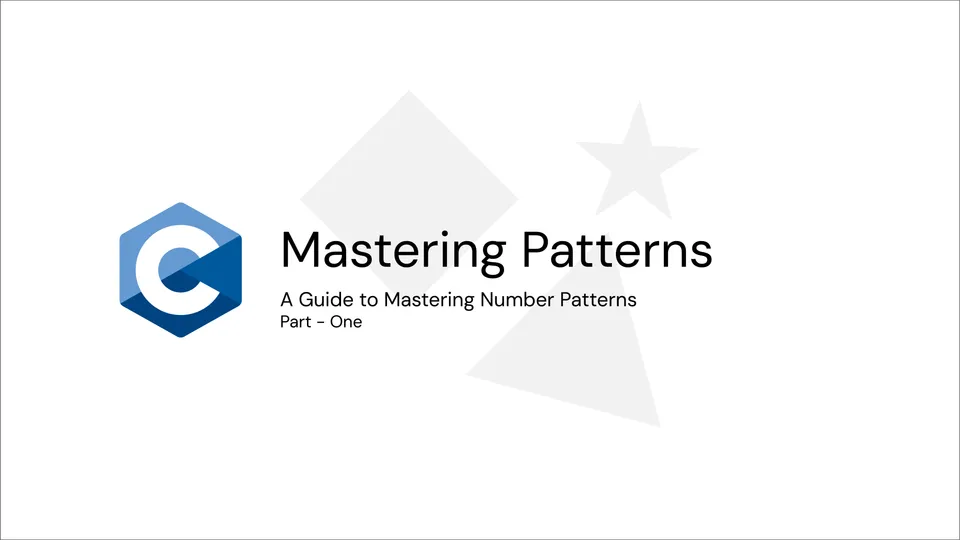
π Pattern Programs in C: A Guide to Mastering Number Patterns Part - One π
Share it on
Hereβs the enhanced blog post with emojis to make it more engaging:
Creating pattern programs is a fantastic way to understand looping and control structures in programming! Here, weβll dive into five different pattern programs in C with step-by-step explanations, examples, and outputs. These will help you master nested loops and pattern techniques in C.
1. πΊ Increasing Triangle Pattern
Code
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number : ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= i; j++)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation
This program takes an integer input n
and prints a triangle pattern π that starts with 1
at the top and increases incrementally on each line. The outer loop controls the rows, while the inner loop manages the numbers within each row.
π Example and Output
For input n = 5
:
Enter a number : 5
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
2. π» Reverse Triangle Pattern
Code
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number : ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = i; j >= 1; j--)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation
This pattern creates a reverse triangle π½, starting from the row number down to 1
for each row. The inner loop runs in descending order, producing numbers in reverse for each row controlled by the outer loop.
π Example and Output
For input n = 5
:
Enter a number : 5
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
3. πΌ Increasing Bottom-Aligned Triangle
Code
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number : ");
scanf("%d", &n);
for(i = n; i >= 1; i--){
for(j = i; j <= n; j++)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation
This pattern starts from the largest row and works upwards, creating a bottom-aligned triangle β¬οΈ. The inner loop starts from the current row number i
and continues up to n
, filling in rows to form the triangle.
π Example and Output
For input n = 5
:
Enter a number : 5
5
4 5
3 4 5
2 3 4 5
1 2 3 4 5
4. π§ Decreasing Triangle Pattern
Code
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number : ");
scanf("%d", &n);
for(i = n; i >= 1; i--){
for(j = 1; j <= i; j++)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation
This pattern produces a decreasing triangle ποΈ, starting with n
numbers in the first row and reducing by one in each following row. The outer loop iterates from n
to 1
, while the inner loop prints numbers from 1
up to the current row number i
.
π Example and Output
For input n = 5
:
Enter a number : 5
1 2 3 4 5
1 2 3 4
1 2 3
1 2
1
5. β¬οΈ Inverted Right-Angled Triangle
Code
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number : ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = n; j >= i; j--)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation
This pattern creates an inverted right-angled triangle β¬οΈ. It starts with a descending sequence from n
to the current row number, with the outer loop iterating from 1
to n
and the inner loop decrementing from n
to i
for each row.
π Example and Output
For input n = 5
:
Enter a number : 5
5 4 3 2 1
5 4 3 2
5 4 3
5 4
5
These pattern programs provide excellent practice with nested loops and sequencing in C. They not only improve understanding of control structures but also serve as foundational exercises for learning about algorithmic patterns. π Happy coding! π