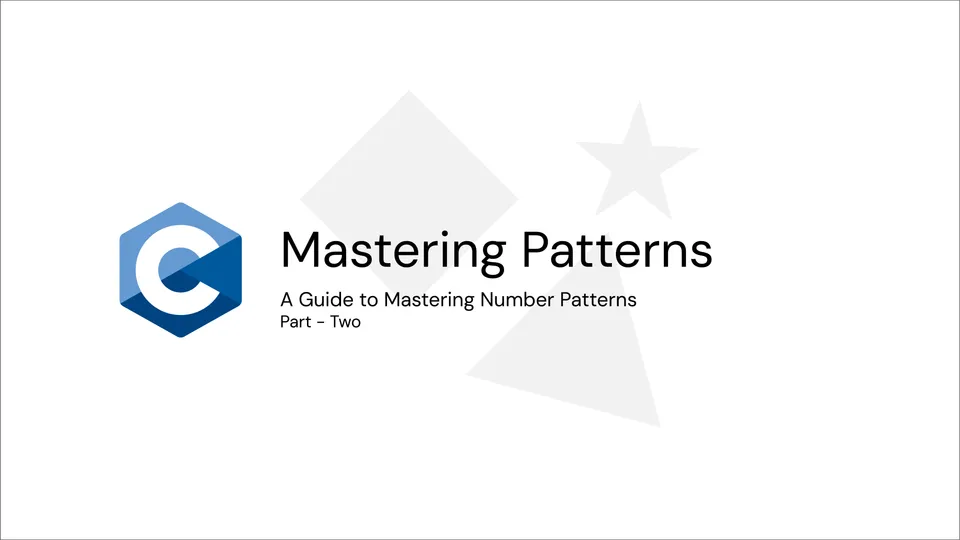
π Pattern Programs in C: A Guide to Mastering Number Patterns Part - Two π
Share it on
Hereβs the updated blog content with some emojis to make it more engaging and visually appealing:
# π 5 Fascinating Pattern Programs in C π
Welcome to a detailed exploration of some popular pattern programs in C. Patterns help solidify our understanding of loops and conditions in programming. Here are five unique patterns you can implement using simple loops in C, along with explanations, examples, and outputs.
---
## 1. β Right-Aligned Star Triangle
This program creates a right-aligned triangle of stars. The number of rows is based on user input, where each row has an increasing number of stars.
### Code:
```c
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number: ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= i; j++)
printf("* ");
printf("\n");
}
return 0;
}
π Explanation:
- Outer Loop (
i
): Controls the rows, ranging from 1 ton
. - Inner Loop (
j
): Prints stars in each row, increasing by one star with each new row.
Example:
If n = 5
, the output will be:
Enter a number: 5
*
* *
* * *
* * * *
* * * * *
2. π’ Binary Triangle (Alternating 1s and 0s by Row)
In this pattern, odd rows contain 1
s, and even rows contain 0
s.
Code:
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number: ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= i; j++){
if(i % 2 == 0)
printf("0 ");
else
printf("1 ");
}
printf("\n");
}
return 0;
}
π Explanation:
- Row Check (
i % 2
): Odd rows print1
, and even rows print0
. - Nested Loop: Prints
i
elements per row based on the current row number.
Example:
If n = 5
, the output will be:
Enter a number: 5
1
0 0
1 1 1
0 0 0 0
1 1 1 1 1
3. π Binary Triangle (Alternating 1s and 0s by Column)
This pattern alternates between 1
and 0
within each row.
Code:
#include<stdio.h>
int main(){
int i, j, n;
printf("Enter a number: ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= i; j++){
if(j % 2 == 0)
printf("0 ");
else
printf("1 ");
}
printf("\n");
}
return 0;
}
π Explanation:
- Column Check (
j % 2
): Alternates values within each row. - Pattern: Begins with
1
for odd columns and0
for even columns.
Example:
If n = 5
, the output will be:
Enter a number: 5
1
1 0
1 0 1
1 0 1 0
1 0 1 0 1
4. π’ Sequential Number Triangle
This pattern increments numbers sequentially across rows, creating a triangle.
Code:
#include<stdio.h>
int main(){
int i, j, n;
int l = 1;
printf("Enter a number: ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= i; j++)
printf("%d ", l++);
printf("\n");
}
return 0;
}
π Explanation:
- Counter
l
: Starts at 1 and increments with each printed number. - Triangle Formation: Each row has one more element than the last.
Example:
If n = 5
, the output will be:
Enter a number: 5
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
5. πΊ Pyramid of Increasing Numbers in Each Row
This program creates a pyramid where each row contains increasing numbers starting from 1, with rows containing odd counts of numbers (1, 3, 5, etc.).
Code:
#include<stdio.h>
int main(){
int i, j, n;
int l = 1;
printf("Enter a number: ");
scanf("%d", &n);
for(i = 1; i <= n; i++){
for(j = 1; j <= 2 * i - 1; j++)
printf("%d ", j);
printf("\n");
}
return 0;
}
π Explanation:
- Inner Loop Condition: Each row has
2 * i - 1
numbers, which aligns with the row structure in a pyramid. - Row Numbers: Restart at
1
in each row and increase sequentially.
Example:
If n = 5
, the output will be:
Enter a number: 5
1
1 2 3
1 2 3 4 5
1 2 3 4 5 6 7
1 2 3 4 5 6 7 8 9
Each of these pattern programs in C showcases different aspects of looping and control structures. These patterns are great exercises for mastering loops, conditional logic, and understanding the flow of control in programming. Happy Coding! π