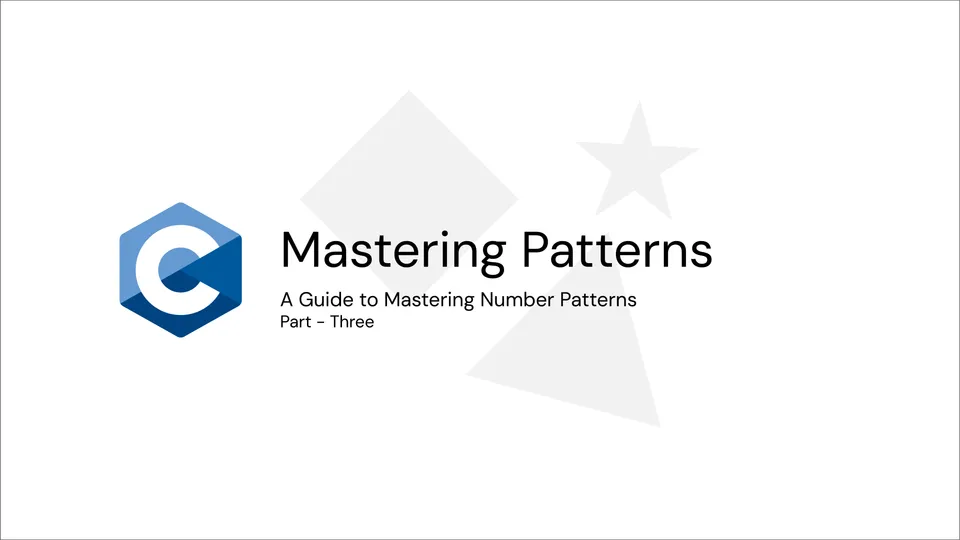
π Pattern Programs in C: A Guide to Mastering Number Patterns Part - Three π
Share it on
Five Pattern Programs in C π
Patterns are a great way to understand looping and nested structures in programming. Here, weβll explore five different pattern programs in C, each demonstrating various ways of structuring loops. These examples will help you grasp key concepts of nested loops, conditional logic, and output formatting in C. Letβs get started! π
1. Right-Aligned Number Pyramid π
This program creates a right-aligned number pyramid. The numbers increase from 1
to the row number in each row, aligning to the right.
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number : ");
scanf("%d", &n);
for (i = 1; i <= n; i++) {
for (l = 1; l <= n - i; l++)
printf(" ");
for (j = 1; j <= i; j++)
printf("%d", j);
printf("\n");
}
return 0;
}
Explanation
- The outer loop (
i
) represents each row. - The first inner loop (
l
) handles spaces to right-align the numbers. - The second inner loop (
j
) prints numbers from1
toi
.
Example Output
For n = 5
, the output will be:
1
12
123
1234
12345
2. Right-Aligned Inverted Pyramid β¬οΈ
This program creates a right-aligned inverted number pattern starting from the input number n
and decreasing each row.
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number : ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
for (l = 1; l <= i - 1; l++)
printf(" ");
for (j = i; j <= n; j++)
printf("%d", j);
printf("\n");
}
return 0;
}
Explanation
- The outer loop (
i
) decreases with each row, starting fromn
. - The first inner loop (
l
) manages spaces to right-align the numbers. - The second inner loop (
j
) prints numbers fromi
ton
.
Example Output
For n = 5
, the output will be:
5
45
345
2345
12345
3. Left-Aligned Descending Pyramid π»
In this pattern, numbers start from the current row number and decrease until reaching the base of the pyramid.
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number : ");
scanf("%d", &n);
for (i = 1; i <= n; i++) {
for (l = 1; l <= i - 1; l++)
printf(" ");
for (j = i; j <= n; j++)
printf("%d", j);
printf("\n");
}
return 0;
}
Explanation
- The outer loop (
i
) iterates for each row. - The first inner loop (
l
) controls spaces at the beginning of each row. - The second inner loop (
j
) prints numbers fromi
ton
, shifting left each row.
Example Output
For n = 5
, the output will be:
12345
2345
345
45
5
4. Left-Aligned Inverted Pyramid πΌ
This program produces a left-aligned inverted pyramid where each row begins with 1
and the number count decreases until n
reaches 1
.
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number : ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
for (l = 1; l <= n - i; l++)
printf(" ");
for (j = 1; j <= i; j++)
printf("%d", j);
printf("\n");
}
return 0;
}
Explanation
- The outer loop (
i
) decreases row by row. - The first inner loop (
l
) manages spaces to align the numbers to the left. - The second inner loop (
j
) prints numbers from1
toi
.
Example Output
For n = 5
, the output will be:
12345
1234
123
12
1
5. Center-Aligned 1 Pyramid π
In this pattern, the program creates a centered pyramid of 1
s, with spaces between each 1
.
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number : ");
scanf("%d", &n);
for (i = 1; i <= n; i++) {
for (l = 1; l <= n - i; l++)
printf(" ");
for (j = 1; j <= i; j++)
printf("1 ");
printf("\n");
}
return 0;
}
Explanation
- The outer loop (
i
) represents rows, with the total number of rows equal ton
. - The first inner loop (
l
) creates spaces for centering the1
s. - The second inner loop (
j
) prints1
with a space in between for each column.
Example Output
For n = 5
, the output will be:
1
1 1
1 1 1
1 1 1 1
1 1 1 1 1
These patterns provide insights into using nested loops, alignment with spaces, and the logic for generating pyramids and inverted pyramids. Try experimenting with different values of n
to see how the patterns change! π§©
Happy Coding! π