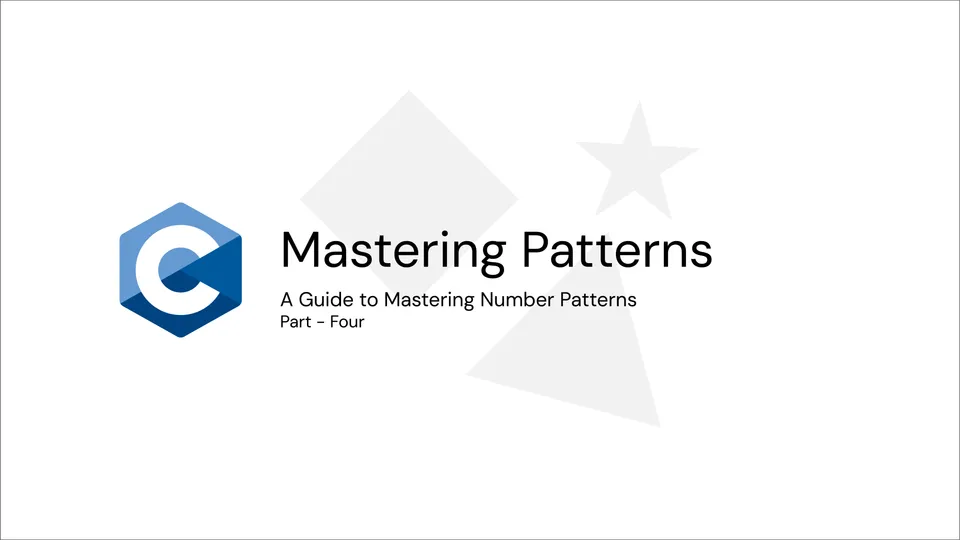
π Pattern Programs in C: A Guide to Mastering Number Patterns Part - Four π
Share it on
Pattern Programs in C
In this blog, we will explore five interesting pattern programs in C. These programs utilize loops and conditional statements to create various number patterns based on user input. Letβs dive into each program and understand how they work, along with example outputs.
1. Inverted Right-Angled Triangle
#include <stdio.h>
int main() {
int i, j, l, n;
printf("Enter a number: ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
for (l = 1; l <= n - i; l++)
printf(" ");
for (j = i; j <= 2 * i - 1; j++)
printf("%d", j);
printf("\n");
}
return 0;
}
Explanation
This program prints an inverted right-angled triangle pattern of numbers. The outer loop iterates from n
down to 1
, controlling the number of rows. The first inner loop prints spaces for formatting, and the second inner loop prints the numbers starting from i
up to 2*i - 1
.
Example Output
Enter a number: 5
56789
4567
345
23
1
2. Pyramid of Numbers
#include <stdio.h>
int main() {
int i, j, k, l, n;
printf("Enter a number: ");
scanf("%d", &n);
for (i = 1; i <= n; i++) {
l = 1;
for (k = 1; k <= n - i; k++)
printf(" ");
for (j = 1; j <= 2 * i - 1; j++) {
printf("%d", l);
if (j < i)
l++;
else
l--;
}
printf("\n");
}
return 0;
}
Explanation
This program prints a pyramid pattern where the numbers increase to the middle and then decrease. The outer loop iterates from 1
to n
, while the first inner loop manages the spaces. The second inner loop prints the numbers, increasing until the midpoint and decreasing afterward.
Example Output
Enter a number: 5
1
121
12321
1234321
123454321
3. Inverted Pyramid with Descending Numbers
#include <stdio.h>
int main() {
int i, j, k, l, n;
printf("Enter a number: ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
l = n - i + 1;
for (k = 1; k <= l - 1; k++)
printf(" ");
for (j = 1; j <= 2 * i - 1; j++) {
printf("%d", l);
if (j < i)
l++;
else
l--;
}
printf("\n");
}
return 0;
}
Explanation
This program produces an inverted pyramid pattern with descending numbers. The outer loop iterates from n
to 1
, determining the number of rows. The first inner loop prints leading spaces, while the second inner loop controls the number output, similar to the previous pyramid pattern but starting from l
.
Example Output
Enter a number: 5
54321
4444
333
22
1
4. Inverted Pyramid with Ascending Numbers
#include <stdio.h>
int main() {
int i, j, k, l, n;
printf("Enter a number: ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
l = 1;
for (k = 1; k <= n - i; k++)
printf(" ");
for (j = 1; j <= 2 * i - 1; j++) {
printf("%d", l);
if (j < i)
l++;
else
l--;
}
printf("\n");
}
return 0;
}
Explanation
This program also prints an inverted pyramid, but it displays 1
s in the first column and increases the number with each step to the center before decreasing. The structure of the loops is similar to the previous patterns.
Example Output
Enter a number: 5
11111
2222
333
44
5
5. Diamond Pattern with Spaces
#include <stdio.h>
int main() {
int i, j, l, k, n;
printf("Enter a number: ");
scanf("%d", &n);
for (i = n; i >= 1; i--) {
l = 1;
k = i;
for (j = 1; j <= 2 * n - 1; j++) {
if (l <= i)
printf("%d", l);
else
printf(" ");
if (j < n)
l++;
else
l--;
}
printf("\n");
}
return 0;
}
Explanation
This program creates a diamond-like shape where the numbers start from 1
and increment outward, then decrement back to 1
. The outer loop iterates from n
down to 1
, while the inner loops manage the spacing and number printing.
Example Output
Enter a number: 5
12345
1234
123
12
1
These pattern programs illustrate how to use loops and conditions effectively in C programming. Each pattern serves as a good exercise for beginners to enhance their understanding of nested loops and formatting outputs.