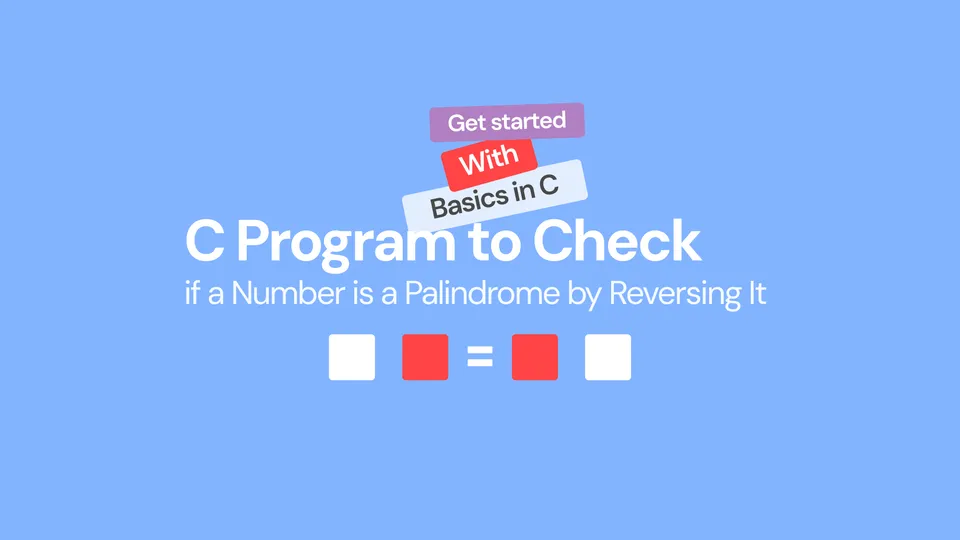
Basics in C: C Program to Check if a Number is a Palindrome by Reversing It
Share it on
π Description:
In this blog post, weβll explore a simple yet effective C program that reverses a given number and checks whether it is a palindrome. A palindrome is a number that reads the same forward and backward, such as 121 or 12321. This program helps you determine if a number is a palindrome using basic concepts like loops, conditional statements, and arithmetic operations.
π Code Implementation:
#include<stdio.h>
int main(){
int n,s,m;
s=0;
printf("Enter a number : ");
scanf("%d", &n);
m=n;
while (n>0){
s=s*10+n%10;
n=n/10;
}
printf("Reverse of the number is %d\n", s);
(s==m)?printf("It is a palindrome number...\n"):printf("Not a palindrome number...\n");
return 0;
}
π Explanation:
- Input: The program prompts the user to enter a number.
- Reversal Process: The entered number is reversed using a
while
loop. - Palindrome Check: After reversing, the program compares the reversed number with the original number.
- Output: It prints whether the number is a palindrome or not.
π Examples:
Example 1:
- Input:
121
- Process:
- Reversed Number:
121
- Since the reversed number is equal to the original number,
121
is a palindrome.
- Reversed Number:
- Output:
Enter a number: 121 Reverse of the number is 121 It is a palindrome number...
Example 2:
- Input:
1234
- Process:
- Reversed Number:
4321
- Since the reversed number is not equal to the original number,
1234
is not a palindrome.
- Reversed Number:
- Output:
Enter a number: 1234 Reverse of the number is 4321 Not a palindrome number...
π― Conclusion:
This simple program helps in determining if a number is a palindrome by reversing it. Palindrome numbers have interesting properties and are often used in various programming challenges. Try this code with different inputs and see the results! Happy coding! π