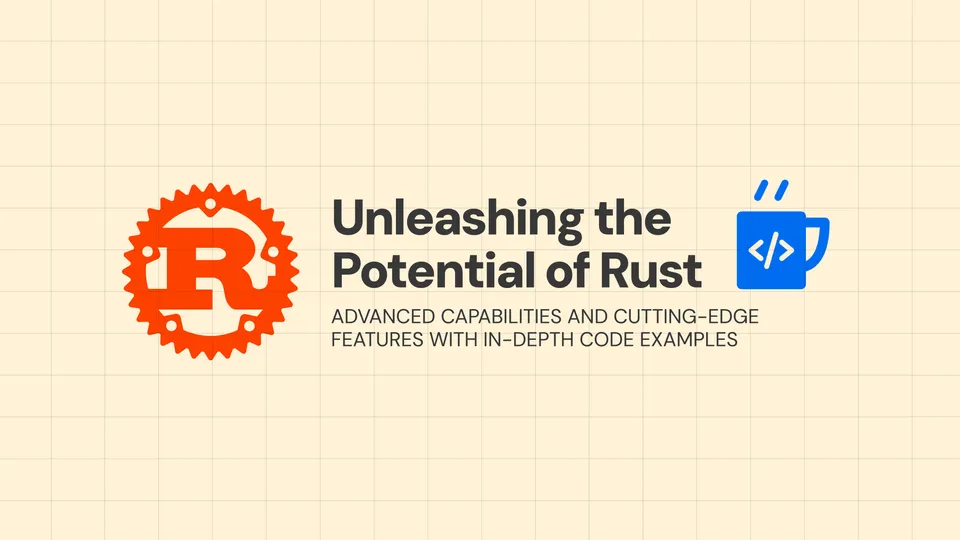
๐ Unleashing the Potential of Rust: Advanced Capabilities and Cutting-Edge Features with In-Depth Code Examples
Share it on
๐ Introduction
Rust, an avant-garde systems programming language renowned for its performance, reliability, and productivity, continues to make significant strides in the software development landscape. In this blog, we will explore Rustโs core capabilities, delve into its latest features, and present intricate code examples that demonstrate its power and versatility.
๐ What is Rust?
Rust is a pioneering programming language designed to offer memory safety without sacrificing performance. It aims to facilitate the development of robust and efficient software through its unique ownership system and strong type guarantees.
๐ ๏ธ Core Capabilities of Rust
- ๐ง Memory Safety: Rustโs ownership system, combined with its strict compile-time checks, ensures memory safety by eliminating data races and null pointer dereferences without the overhead of a garbage collector.
- โก Concurrency: Rustโs concurrency model, built around ownership and type safety, provides robust concurrency primitives that enable developers to write safe and performant concurrent code.
- ๐ Zero-Cost Abstractions: Rustโs abstractions, from iterators to smart pointers, are designed to incur no runtime overhead, ensuring that high-level code remains as efficient as hand-written low-level code.
- ๐งฉ Pattern Matching: Rustโs pattern matching is both expressive and powerful, allowing for concise and readable code when handling complex data structures.
- ๐ Interoperability: Rust seamlessly integrates with C and other languages, making it an ideal choice for a wide range of development needs, from systems programming to web development.
๐ Innovative New Features in Rust
๐งฎ 1. Const Generics
Const generics introduce greater flexibility and power to generic programming in Rust by allowing generic parameters to be constants, enabling more expressive and reusable code.
fn create_matrix<T, const ROWS: usize, const COLS: usize>(value: T) -> [[T; COLS]; ROWS]
where
T: Clone + Default,
{
[[value; COLS]; ROWS]
}
fn main() {
let matrix = create_matrix(0, 3, 4);
println!("{:?}", matrix);
}
โ๏ธ 2. Enhanced Async/Await
Rustโs async/await syntax has been further refined, enhancing ergonomic and efficient asynchronous programming, allowing for seamless handling of asynchronous operations.
use tokio::time::{sleep, Duration};
async fn fetch_data() -> &'static str {
sleep(Duration::from_secs(2)).await;
"Data retrieved"
}
#[tokio::main]
async fn main() {
let data = fetch_data().await;
println!("{}", data);
}
๐ ๏ธ 3. Inline Assembly
The introduction of inline assembly in Rust allows developers to write low-level assembly code within Rust programs, providing precise control over performance-critical sections.
fn main() {
let result: u64;
unsafe {
asm!(
"mov {0}, 42",
out(reg) result
);
}
println!("Result: {}", result);
}
๐ 4. Track Caller Attribute
The #[track_caller]
attribute enhances error reporting by providing more detailed context when panics occur, aiding in debugging and improving the developer experience.
#[track_caller]
fn assert_non_negative(value: i32) {
if value < 0 {
panic!("Negative value: {}", value);
}
}
fn main() {
assert_non_negative(-1);
}
๐ Real-World Example: Building an Advanced Web Server with Rust
To demonstrate Rustโs capabilities, letโs construct a sophisticated web server using the Actix web framework, complete with route handling and database integration.
use actix_web::{web, App, HttpServer, Responder, HttpResponse};
use serde::{Deserialize, Serialize};
use sqlx::{PgPool, query_as};
#[derive(Serialize, Deserialize)]
struct Data {
id: i32,
name: String,
value: String,
}
async fn get_data(pool: web::Data<PgPool>) -> impl Responder {
let data: Vec<Data> = query_as!(
Data,
"SELECT id, name, value FROM data_table"
)
.fetch_all(pool.get_ref())
.await
.expect("Failed to fetch data");
HttpResponse::Ok().json(data)
}
async fn greet() -> impl Responder {
HttpResponse::Ok().body("Hello, Rust!")
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
let pool = PgPool::connect("postgres://user:password@localhost/database").await.unwrap();
HttpServer::new(move || {
App::new()
.data(pool.clone())
.route("/hello", web::get().to(greet))
.route("/data", web::get().to(get_data))
})
.bind("127.0.0.1:8080")?
.run()
.await
}
๐ Conclusion
Rustโs advanced capabilities and the continuous introduction of innovative features make it a formidable choice for modern software development. From ensuring memory safety and leveraging robust concurrency models to embracing zero-cost abstractions and seamless interoperability, Rust offers a powerful toolkit for developers. By harnessing these features, developers can craft efficient, reliable, and maintainable code, positioning Rust as a language for the future.