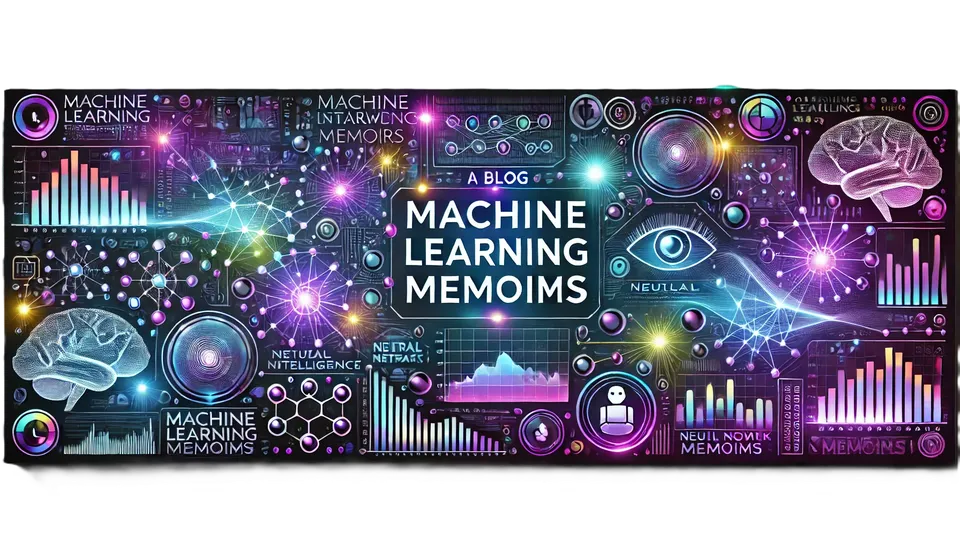
Machine Learning Memoirs: Your Friendly Guide to ML Magic! 🚀
Share it on
Machine Learning isn’t just a buzzword; it’s the wizardry behind everything cool in tech—think self-driving cars, chatbots, and even Netflix knowing exactly what you want to binge next. 😄 Whether you’re just dipping your toes into the ML waters or ready to ride the wave, this post is here to make learning fun, approachable, and full of aha moments! 🌟
🤔 So, What is Machine Learning?
Imagine teaching your computer to learn just like you do! ML is all about creating algorithms that analyze data, spot patterns, and make decisions with minimal human input.
- Supervised Learning: You’re the teacher! Provide labeled data, and the algorithm learns from it.
- Unsupervised Learning: No labels? No problem! The algorithm explores patterns on its own.
- Reinforcement Learning: Think trial and error—like training a doggo to fetch treats. 🐾
⚡️ Let’s Get Our Hands Dirty with Code!
Before we start, make sure you’ve got the tools ready. Install these libraries if you haven’t already:
pip install numpy pandas matplotlib scikit-learn
📈 Example 1: Predict the Future with Linear Regression
Linear regression is like drawing a straight line through a cloud of points to predict future values. Let’s build one!
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error, r2_score
import matplotlib.pyplot as plt
# Generating some random data 🎲
np.random.seed(42)
X = np.random.rand(100, 1) * 10 # Feature: random numbers between 0 and 10
y = 3 * X + 7 + np.random.randn(100, 1) # Target: y = 3X + 7 + noise
# Splitting data into training and testing 🛠️
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Training the model 🤖
model = LinearRegression()
model.fit(X_train, y_train)
# Making predictions 🔮
y_pred = model.predict(X_test)
# Evaluating the model 📊
mse = mean_squared_error(y_test, y_pred)
r2 = r2_score(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
print(f"R^2 Score: {r2}")
# Visualizing the results 🎨
plt.scatter(X_test, y_test, color='blue', label='Actual')
plt.plot(X_test, y_pred, color='red', label='Predicted')
plt.xlabel('Feature (X)')
plt.ylabel('Target (y)')
plt.title('Linear Regression: Actual vs Predicted')
plt.legend()
plt.show()
💡 Cool Fact: This type of regression is widely used in financial predictions and even in real estate pricing!
🎨 Example 2: Finding Hidden Groups with K-Means Clustering
Let’s say you have a bunch of unorganized data—how do you group it into something meaningful? Enter K-Means Clustering, the ultimate party organizer! 🎉
from sklearn.cluster import KMeans
from sklearn.datasets import make_blobs
import matplotlib.pyplot as plt
# Creating some sample blobs 💡
X, _ = make_blobs(n_samples=300, centers=4, cluster_std=0.6, random_state=0)
# Applying K-Means 🎯
kmeans = KMeans(n_clusters=4)
kmeans.fit(X)
# Getting cluster centers and labels
centroids = kmeans.cluster_centers_
labels = kmeans.labels_
# Visualizing the clusters 🌈
plt.scatter(X[:, 0], X[:, 1], c=labels, cmap='rainbow', s=50, alpha=0.7, label='Data Points')
plt.scatter(centroids[:, 0], centroids[:, 1], color='black', s=200, marker='X', label='Centroids')
plt.title('K-Means Clustering')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
plt.legend()
plt.show()
💡 Cool Fact: K-Means is often used in customer segmentation, so businesses know who loves coffee and who’s there for tea! ☕
🌳 Example 3: Making Decisions with Decision Trees
Decision Trees are like a flowchart of choices—perfect for breaking down complex decisions! Let’s try it with the famous Iris dataset. 🌺
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier, plot_tree
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Loading the Iris dataset 🌼
iris = load_iris()
X = iris.data
y = iris.target
# Splitting into training and testing 🤹
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Training the Decision Tree 🌳
tree_model = DecisionTreeClassifier(max_depth=3, random_state=42)
tree_model.fit(X_train, y_train)
# Making predictions 📊
y_pred = tree_model.predict(X_test)
# Checking accuracy 🏆
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy * 100:.2f}%")
# Visualizing the Decision Tree 🎨
plt.figure(figsize=(12, 8))
plot_tree(tree_model, filled=True, feature_names=iris.feature_names, class_names=iris.target_names)
plt.show()
💡 Cool Fact: Decision Trees power recommendation systems—like suggesting your next favorite playlist on Spotify! 🎵
✨ Pro Tips for Aspiring ML Wizards
- Play with Data! 🏀 The more you practice, the better you’ll understand patterns and anomalies.
- Keep it Simple. 🧩 Start with easy algorithms like Linear Regression before diving into Neural Networks.
- Experimentation = Growth. 🌱 Try different datasets, tweak parameters, and see how the results change.
Wrapping it Up with a Bow 🎀
You’ve just scratched the surface of the magical world of Machine Learning! 🌌 Whether you’re predicting trends, organizing data, or making smarter decisions, ML opens up endless possibilities. With each experiment, you’re one step closer to building something extraordinary. 🚀
Now go forth and code! The world is your dataset. 🌍