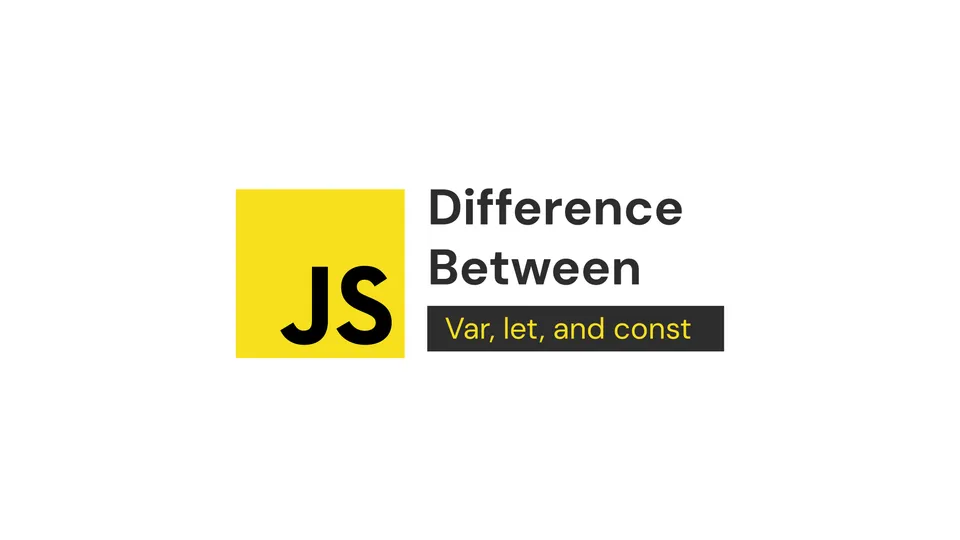
In JavaScript, we can declare variables using three keywords:
1. var
When a variable is declared using var
, it means it is globally scoped.
var x = 1;
if (x === 1) {
var x = 2;
// Expected Output: 2
console.log(x);
}
// Expected Output: 2
console.log(x);
2. let
If we declare a variable using let
, it is a locally scoped variable.
let a = 1;
if (a === 1) {
let a = 2;
// Expected Output: 2
console.log(a);
}
// Expected Output: 1
console.log(a);
3. const
When a variable is declared as const
, it means throughout the program the value of the variable will remain the same. It means the value will never be changed if a variable is declared as const
.
const num = 10;
num = 100;
console.log(num);
// Expected output: TypeError: Assignment to constant variable.