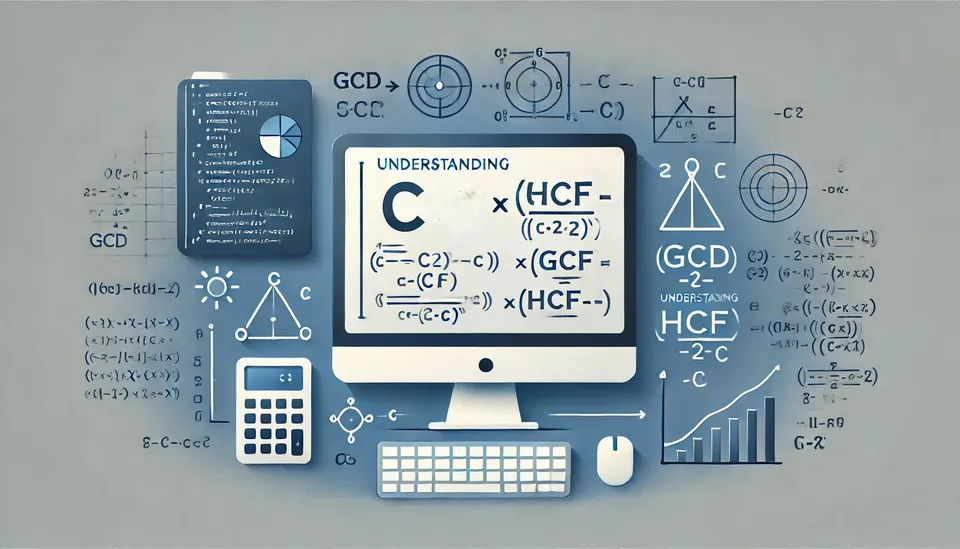
Finding the Highest Common Factor (HCF), also known as the Greatest Common Divisor (GCD), of two numbers is a fundamental concept in mathematics. In this blog, weβll explore how to compute the HCF using C programming with the help of the Euclidean algorithm. π
Introduction π
The HCF of two integers is the largest positive integer that divides both numbers without leaving a remainder. Itβs a handy concept used in fractions, ratios, and number theory.
In this blog, weβll dive into a simple C program that calculates the HCF of two numbers. This program is based on the Euclidean algorithm, which is both efficient and elegant. π
Program Code π¨βπ»
#include<stdio.h>
int main(){
int a,b,r;
printf("Enter two numbers : ");
scanf("%d",&a);
scanf("%d",&b);
do{
r=a%b;
if(r!=0){
a=b;
b=r;
}
}while(r!=0);
printf("HCF of two numbers is %d", b);
return 0;
}
Explanation of the Logic π§
-
Input Prompt:
- The program starts by asking the user to enter two integers. βοΈ
- Example: If the user inputs
36
and60
, these values are stored in the variablesa
andb
respectively.
-
Euclidean Algorithm:
- The algorithm repeatedly replaces the larger number with the remainder of dividing the larger number by the smaller number. π
- Letβs break it down for
36
and60
:- First iteration:
r = 36 % 60 = 36
. Updatea = 60
,b = 36
. - Second iteration:
r = 60 % 36 = 24
. Updatea = 36
,b = 24
. - Third iteration:
r = 36 % 24 = 12
. Updatea = 24
,b = 12
. - Fourth iteration:
r = 24 % 12 = 0
. The loop stops here. β
- First iteration:
- The value of
b
(12 in this case) is the HCF. π
-
Output:
- The program displays the calculated HCF using a
printf
statement. π¨οΈ
- The program displays the calculated HCF using a
Sample Output π
Hereβs an example of the program in action:
Input:
Enter two numbers: 36 60
Output:
HCF of two numbers is 12
Conclusion π
The program above demonstrates how to calculate the HCF of two numbers using the Euclidean algorithm. This method is simple, effective, and perfect for handling even large numbers. With this understanding, you can now incorporate HCF calculation into your own C programs for a variety of applications. π‘
Give it a try and experiment with different pairs of numbers to see the magic of the algorithm in action! β¨