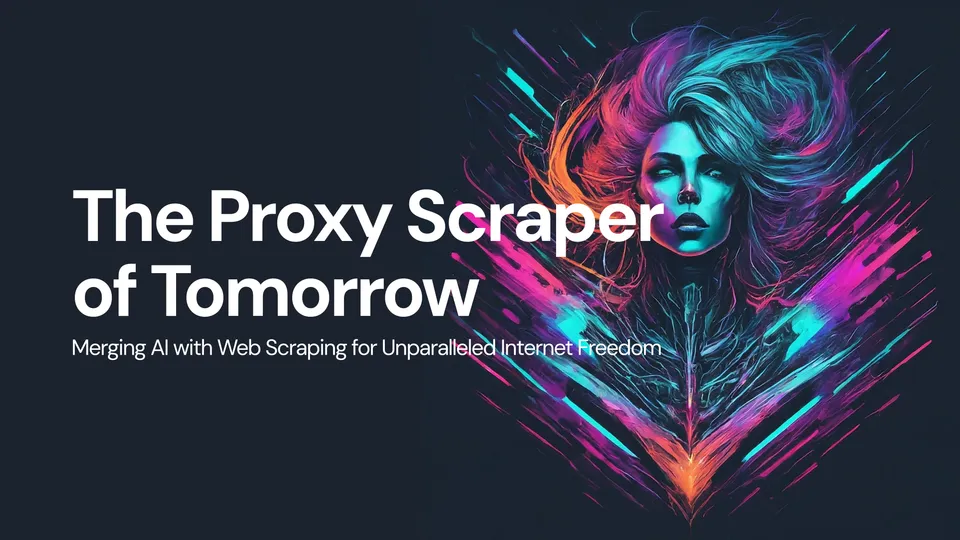
🌐 The Proxy Scraper of Tomorrow: Merging AI with Web Scraping for Unparalleled Internet Freedom 🚀
Share it on
🤖 Introduction
The internet is an ever-expanding universe, brimming with data, information, and opportunities. But in a world where access is increasingly restricted, proxy scrapers have become the gatekeepers, ensuring anonymity and accessibility. Traditionally, these tools were basic scripts that gathered proxy lists, but as the digital landscape grows more complex, the need for smarter, more adaptable tools is evident. Enter the next generation of proxy scrapers—powered by artificial intelligence and machine learning—designed not just to gather proxies but to analyze, predict, and adapt in real time. 🤖🔍
This blog dives into the architecture of a futuristic proxy scraper, a tool that embodies the cutting edge of technology to navigate the evolving internet. This isn’t just a scraper; it’s a dynamic, AI-driven system that learns and adapts, setting a new standard for proxy scraping. 🌟
🔍 Why Proxy Scraping Needs to Evolve
Proxy scrapers are essential for bypassing geo-restrictions, maintaining anonymity, and ensuring secure connections across the web. However, traditional proxy scrapers face several challenges:
- Inconsistent Reliability: Not all proxies are created equal. Some may be slow, others unreliable, and many might not work at all. 🚫
- Static Lists: Most scrapers work with static lists that quickly become outdated. 📉
- Manual Updates: Updating proxies and maintaining their reliability requires constant manual intervention. 🛠️
The future of proxy scraping lies in automation, intelligence, and adaptability. By integrating AI, we can create a proxy scraper that not only gathers proxies but also evaluates and predicts their reliability, adapting to changes in real-time. 🌐✨
🛠️ The Blueprint of a Futuristic Proxy Scraper
To build this advanced proxy scraper, we’ll leverage Ruby on Rails as the framework, incorporating TensorFlow for machine learning, and Sidekiq for background processing. The goal is to create a scraper that autonomously identifies and ranks proxies, ensuring you always have access to the best available options. 🏆
📂 Step 1: Setting Up the Rails Environment
Start by setting up a new Rails application and installing the necessary gems:
rails new AIProxyScraper
cd AIProxyScraper
# Add necessary gems in Gemfile
gem 'httparty'
gem 'nokogiri'
gem 'tensorflow', '~> 0.3.2'
gem 'sidekiq'
bundle install
These gems will enable HTTP requests, HTML parsing, and AI model integration—essential components for our smart scraper. 🔧
🤖 Step 2: Creating the Proxy Model
We need a database model to store proxies and their AI-predicted reliability scores:
rails generate model Proxy ip:string port:integer protocol:string country:string reliability:float
rails db:migrate
This model will store crucial information about each proxy, including its IP address, port, protocol, country, and an AI-predicted reliability score. 📈
🧠 Step 3: Integrating AI with TensorFlow
Here’s where things get exciting. We’ll build a simple AI model using TensorFlow to predict the reliability of proxies based on historical data. The AI model will learn from past interactions to improve its predictions over time.
Create a new service for the AI model:
mkdir app/services
touch app/services/proxy_model.rb
In app/services/proxy_model.rb
, add the following code:
require 'tensorflow'
class ProxyModel
def initialize
@model = TensorFlow::Graph.new
create_model
end
def create_model
@model.as_default do
@input = TensorFlow::Placeholder.new(TensorFlow::FLOAT32, shape: [-1, 3])
@labels = TensorFlow::Placeholder.new(TensorFlow::FLOAT32, shape: [-1, 1])
dense1 = TensorFlow::Layers::Dense.new(@input, units: 64, activation: :relu)
dense2 = TensorFlow::Layers::Dense.new(dense1, units: 64, activation: :relu)
@output = TensorFlow::Layers::Dense.new(dense2, units: 1, activation: :sigmoid)
@loss = TensorFlow::Losses.mean_squared_error(@labels, @output)
@optimizer = TensorFlow::Train::AdamOptimizer.new(learning_rate: 0.001).minimize(@loss)
end
end
def train(input_data, label_data, epochs = 1000)
input_tensor = TensorFlow::Tensor.new(input_data)
label_tensor = TensorFlow::Tensor.new(label_data)
epochs.times do
_, loss_value = @optimizer.run(@input => input_tensor, @labels => label_tensor)
puts "Training Loss: #{loss_value}" if epochs % 100 == 0
end
end
def predict(input_data)
input_tensor = TensorFlow::Tensor.new(input_data)
@output.run(@input => input_tensor)
end
end
This AI model is the brain behind our proxy scraper. It takes in data (IP address, port, protocol) and outputs a reliability score, helping to weed out unreliable proxies before they’re used. 🧠🔍
🌐 Step 4: Building the Proxy Scraper
Now, we’ll build the actual scraper, integrating it with our AI model to evaluate proxies on the fly:
touch app/services/proxy_scraper.rb
In app/services/proxy_scraper.rb
, add the following code:
require 'httparty'
require 'nokogiri'
class ProxyScraper
BASE_URL = "https://www.sslproxies.org/" # Example proxy list website
def initialize
@model = ProxyModel.new
end
def scrape_proxies
response = HTTParty.get(BASE_URL)
parsed_page = Nokogiri::HTML(response.body)
parsed_page.css('table tbody tr').each do |row|
ip = row.css('td')[0].text.strip
port = row.css('td')[1].text.strip.to_i
protocol = row.css('td')[6].text.strip.downcase
country = row.css('td')[3].text.strip
reliability = predict_reliability(ip, port, protocol)
if reliability > 0.5 # Example threshold
Proxy.create(ip: ip, port: port, protocol: protocol, country: country, reliability: reliability)
end
end
end
def predict_reliability(ip, port, protocol)
input_data = [[ip_to_numeric(ip), port, protocol_to_numeric(protocol)]]
result = @model.predict(input_data)
result[0][0]
end
private
def ip_to_numeric(ip)
ip.split('.').map(&:to_i).inject(0) { |a, b| (a << 8) + b }
end
def protocol_to_numeric(protocol)
case protocol
when 'http'
1
when 'https'
2
else
0
end
end
end
This scraper dynamically fetches proxies, evaluates them using the AI model, and stores only the most reliable ones in the database. It’s a smart, automated solution that ensures your proxy list is always up-to-date and effective. 🚀🔒
⏲️ Step 5: Automating Proxy Testing
To keep the proxy list fresh, we’ll automate the process of testing and updating proxies using Sidekiq. This ensures that the AI model continues to learn and adapt over time:
rails generate job proxy_test
In app/jobs/proxy_test_job.rb
, add:
class ProxyTestJob < ApplicationJob
queue_as :default
def perform
scraper = ProxyScraper.new
scraper.scrape_proxies
proxies = Proxy.all
proxies.each do |proxy|
response_time = test_proxy(proxy.ip, proxy.port)
label = response_time < 1.0 ? 1 : 0 # 1 for reliable, 0 for unreliable
scraper.train([[proxy.ip, proxy.port, proxy.protocol]], [[label]])
end
end
def test_proxy(ip, port)
# Simulate a test to check proxy response time
start_time = Time.now
HTTParty.get("http://example.com", http_proxyaddr: ip, http_proxyport: port)
Time.now - start_time
end
end
This job periodically runs, scraping new proxies, testing them, and feeding the results back into the AI model, which continues to improve and refine its predictions. 🕒🔄
🌍 Step 6: Integrating with the Rails App
To make this scraper accessible, we’ll add routes and a controller to trigger it:
rails generate controller Proxies
In app/controllers/proxies_controller.rb
, add:
class ProxiesController < ApplicationController
def scrape
ProxyTestJob.perform_now
redirect_to proxies_path, notice: "Proxies scraped and tested successfully."
end
def index
@proxies = Proxy.all
end
end
Add routes in config/routes.rb
:
Rails.application.routes.draw do
resources :proxies, only: [:index] do
collection do
get 'scrape'
end
end
root "proxies#index"
end
👁️ Step 7: Displaying Results
Finally, create a simple view to display the proxies and their reliability scores:
In app/views/proxies/index.html.erb
:
<h1>Scraped Proxies</h1>
<%= link_to "Scrape Proxies", scrape_proxies_path, class: "btn btn-primary" %>
<table class="table">
<thead>
<tr>
<th>IP</th>
<th>Port</th>
<th>Protocol</th>
<th>Country</th>
<th>Reliability</th>
</tr>
</thead>
<tbody>
<% @proxies.each do |proxy| %>
<tr>
<td><%= proxy.ip %></td>
<td><%= proxy.port %></td>
<td><%= proxy.protocol %></td>
<td><%= proxy.country %></td>
<td><%= proxy.reliability %></td>
</tr>
<% end %>
</tbody>
</table>
🚀 Running the Application
With everything set up, start your Rails server and navigate to http://localhost:3000/proxies/scrape
to begin scraping proxies and testing their reliability.
rails server
🌟 The Future of Proxy Scraping
This is just the beginning. The proxy scraper we’ve built here represents a new era of web scraping—one where AI and machine learning are at the core, enabling smarter, faster, and more reliable data collection. As the internet continues to evolve, so too will the tools we use to navigate it. By embracing AI and automation, we can ensure that proxy scrapers remain not just relevant, but essential tools for the future. 🌐💡
This fusion of AI with web scraping is more than just a technical advancement; it’s a glimpse into the future of how we interact with and access the internet. The next generation of proxy scrapers will be more than just tools—they will be intelligent systems that understand, adapt, and evolve, ensuring that the internet remains a place of freedom, accessibility, and endless possibility. 🌟🔮