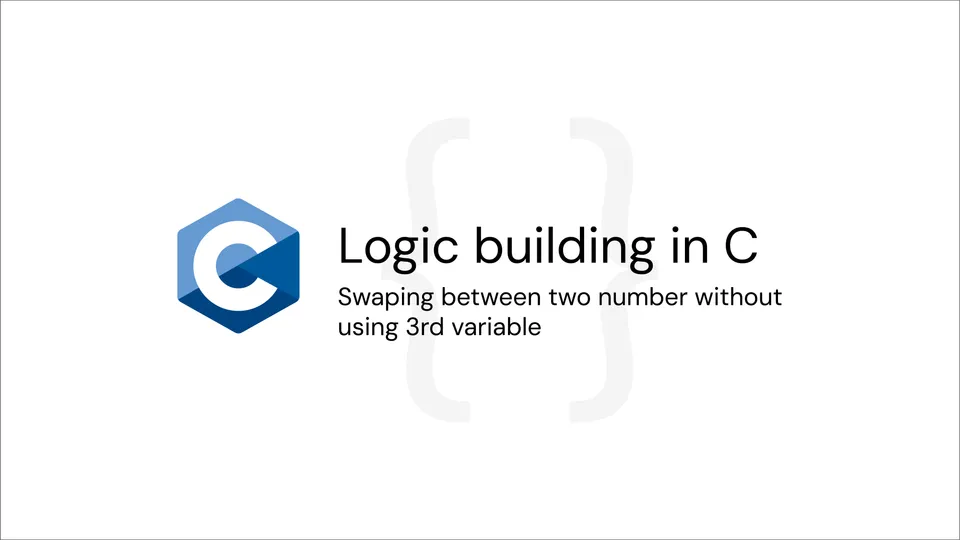
Swapping Variables Without Using a Third Variable in C
Swapping the values of two variables is a common task in programming. A typical approach involves using a third variable to temporarily hold one of the values. However, it is also possible to swap the values without using an additional variable. In this post, we will explore how to achieve this in C.
The Code
Below is the C program to swap two variables without using a third variable:
#include<stdio.h>
int main(){
int a,b;
printf("Enter value for variable A : ");
scanf("%d", &a);
printf("\nEnter value for variable b : ");
scanf("%d", &b);
a = a + b;
b = a - b;
a = a - b;
printf("After swapping...\n");
printf("The value of variable A : %d\nThe value of variable B : %d", a, b);
return 0;
}
Explanation
-
Reading Inputs: The program starts by reading the values of two variables,
a
andb
, from the user.printf("Enter value for variable A : "); scanf("%d", &a); printf("\nEnter value for variable b : "); scanf("%d", &b);
-
Swapping Logic: The core logic of swapping without a third variable involves simple arithmetic operations.
-
First, we add the values of
a
andb
and store the result ina
.a = a + b;
-
Next, we subtract the new value of
a
(which isa + b
) byb
, and store the result inb
. This effectively assigns the original value ofa
tob
.b = a - b;
-
Finally, we subtract the new value of
b
(which is the original value ofa
) froma
, and store the result ina
. This assigns the original value ofb
toa
.a = a - b;
-
-
Output the Results: After the swap, we print the new values of
a
andb
.printf("After swapping...\n"); printf("The value of variable A : %d\nThe value of variable B : %d", a, b);
Example Output
Hereβs an example of how the program works with specific inputs:
Enter value for variable A : 5
Enter value for variable B : 10
After swapping...
The value of variable A : 10
The value of variable B : 5
Conclusion
Swapping two variables without using a third variable is a neat trick that utilizes basic arithmetic operations. This approach is not only efficient but also demonstrates the power of simple mathematical operations in solving problems. Try running the above code with different inputs to see how it works in practice!