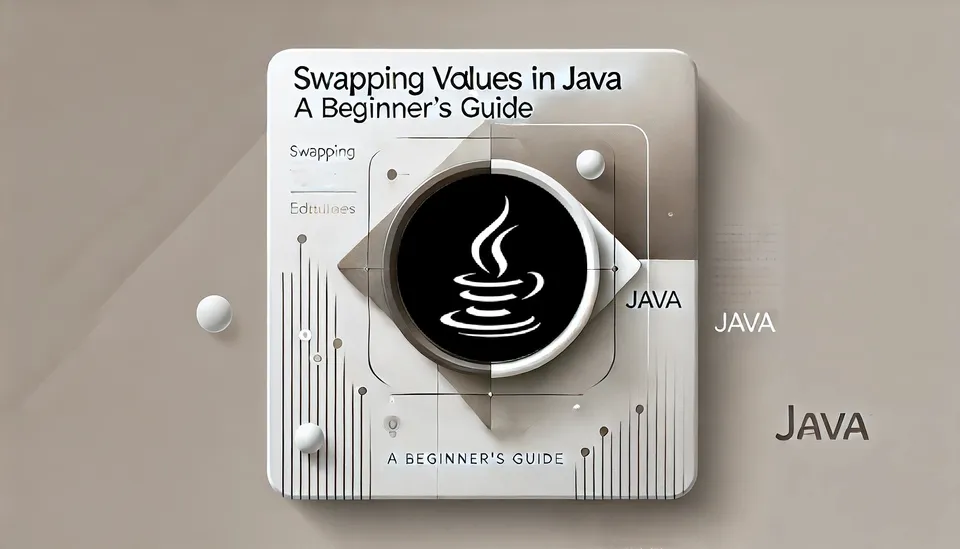
Swapping the values of two variables is a fundamental programming concept. It’s a simple yet essential operation that lays the groundwork for understanding more complex algorithms. In this blog, we’ll explore how to implement a swap operation in Java using a temporary variable.
What Does Swapping Mean?
Swapping refers to exchanging the values of two variables so that the value of one variable is assigned to the other, and vice versa. For instance, if variable a
holds the value 5
and variable b
holds 3
, swapping will result in a
containing 3
and b
containing 5
.
This operation is widely used in sorting algorithms, data manipulation, and other computational processes.
How Does It Work?
The logic for swapping two variables involves using a temporary variable as a placeholder. Here’s the step-by-step process:
- Store the value of the first variable (
a
) in a temporary variable (temp
). - Assign the value of the second variable (
b
) to the first variable (a
). - Retrieve the value stored in the temporary variable (
temp
) and assign it to the second variable (b
).
This ensures that no data is lost during the swap process.
Java Code Implementation
Below is a Java program that demonstrates how to swap the values of two integers using a temporary variable.
public class Swap {
public static void main(String[] args) {
int a, b, temp; // Declare three variables
a = 5; // Initialize a
b = 3; // Initialize b
// Display values before swapping
System.out.println("Before swapping...");
System.out.println("Value of a = " + a);
System.out.println("Value of b = " + b);
// Swap the values using a temporary variable
temp = a;
a = b;
b = temp;
// Display values after swapping
System.out.println("After swapping...");
System.out.println("Value of a = " + a);
System.out.println("Value of b = " + b);
}
}
Output
When you run the program, you’ll see the following output:
Before swapping...
Value of a = 5
Value of b = 3
After swapping...
Value of a = 3
Value of b = 5
Understanding the Logic
Variable Declaration and Initialization:
- Two variables,
a
andb
, are declared and initialized with the values5
and3
, respectively. - A third variable,
temp
, is declared to temporarily hold data during the swap.
Swapping:
- The value of
a
is assigned totemp
, preserving its initial value. - The value of
b
is then assigned toa
, completing half of the swap. - Finally, the preserved value from
temp
is assigned tob
, completing the operation.
Display:
- The program displays the values of
a
andb
before and after the swap for clarity.
Why Use a Temporary Variable?
Using a temporary variable ensures that the original values are not overwritten during the swap. This method is straightforward and ideal for beginners. While there are other swapping techniques, such as using arithmetic operations (addition and subtraction, XOR, etc.), the use of a temporary variable is the most intuitive and least prone to errors.
Conclusion
Swapping values is a simple yet powerful concept in programming. The example above demonstrates the basic principles behind swapping, making it a great starting point for beginners. By understanding and implementing this logic, you can build a strong foundation for more advanced programming tasks.
Feel free to try the program and modify it for different values or variable types!