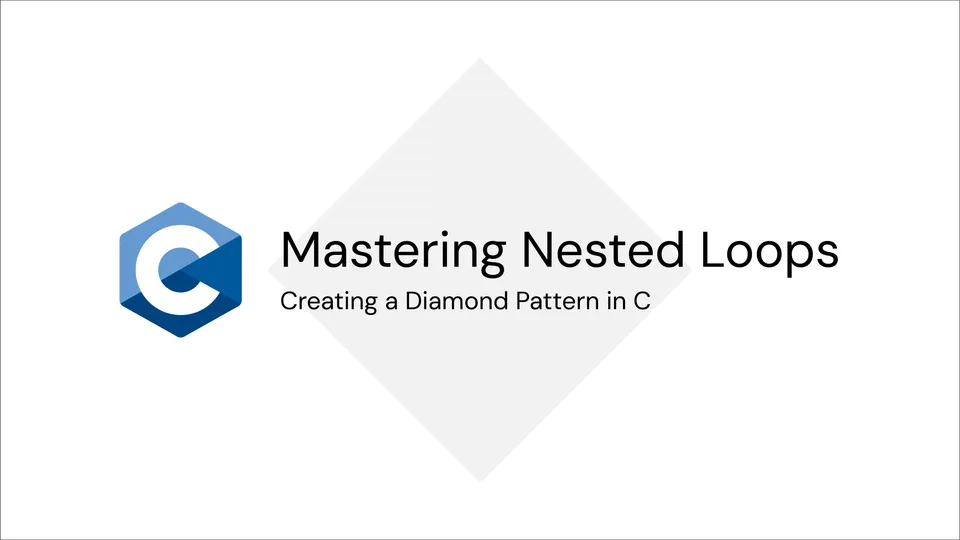
How to solve pattern problems
-
Understand the Pattern: Carefully observe the given pattern. Identify how the rows and columns are structured and note any repeating elements.
-
Identify the Dimensions: Determine the number of rows and columns in the pattern. This helps in setting up the outer and inner loops.
-
Establish the Relationship: Figure out the relationship between the row index and the column index. This often involves understanding how the elements in each row are related to the row number.
-
Write the Loops: Use nested loops to iterate through the rows and columns. The outer loop usually controls the rows, while the inner loop controls the columns.
-
Implement Conditions: Add any necessary conditions inside the loops to print the correct elements. This may involve
if-else
statements to handle different parts of the pattern.
Let’s do a Dimond pattern by using above step’s
-
Variable Declarations:
i, j, k
: Loop counters.n
: The maximum number (input from the user).m
: The total number of rows in the diamond pattern, calculated as2 * n - 1
.l
: Initial number of spaces before the first number in each row.b
: Number of elements (numbers) in the middle section of the diamond pattern.
-
User Input:
- The program prompts the user to enter the maximum number
n
, which defines the size of the diamond.
- The program prompts the user to enter the maximum number
-
Loop Structure:
- The outer loop (
for (i = 1; i <= m; i++)
) iterates over each row. - The first inner loop (
for (k = 1; k <= l; k++)
) prints spaces before the numbers in each row. - The second inner loop (
for (j = 1; j <= 2 * b - 1; j++)
) prints the numbers in each row.
- The outer loop (
-
Number Printing Logic:
- If
j
is greater thanb
, it prints2 * b % j
. - Otherwise, it prints
j
.
- If
-
Adjusting the Pattern:
- If the current row
i
is greater than or equal ton
, it increases the number of spaces (l++
) and decreases the number of elements (b--
). - Otherwise, it decreases the number of spaces (
l--
) and increases the number of elements (b++
).
- If the current row
Code Implementation
Here’s how you can implement this in c:
#include<stdio.h>
int main() {
int i, j, k, n, m, l, b;
printf("Enter the maximum number : ");
scanf("%d", &n);
m = 2 * n - 1;
l = n - 1;
b = 1;
for (i = 1; i <= m; i++) {
for (k = 1; k <= l; k++)
printf(" ");
for (j = 1; j <= 2 * b - 1; j++) {
if (j > b)
printf("%d ", 2 * b % j);
else
printf("%d ", j);
}
if (i >= n) {
l++;
b--;
} else {
l--;
b++;
}
printf("\n");
}
return 0;
}
Example Output
If the user enters n = 4
, the output will be a diamond pattern:
Enter the maximum number : 4
1
1 2 1
1 2 3 2 1
1 2 3 4 3 2 1
1 2 3 2 1
1 2 1
1
Step-by-Step Output Explanation
-
First Row:
- Spaces: 6 (3 * 2)
- Numbers: 1
-
Second Row:
- Spaces: 4 (2 * 2)
- Numbers: 1 2 1
-
Third Row:
- Spaces: 2 (1 * 2)
- Numbers: 1 2 3 2 1
-
Fourth Row:
- Spaces: 0
- Numbers: 1 2 3 4 3 2 1
-
Fifth Row (mirror of the third row):
- Spaces: 2
- Numbers: 1 2 3 2 1
-
Sixth Row (mirror of the second row):
- Spaces: 4
- Numbers: 1 2 1
-
Seventh Row (mirror of the first row):
- Spaces: 6
- Numbers: 1
This pattern is created by first building the top half of the diamond, then mirroring it to form the bottom half. The code adjusts the spaces and number of elements accordingly to achieve this symmetrical pattern.