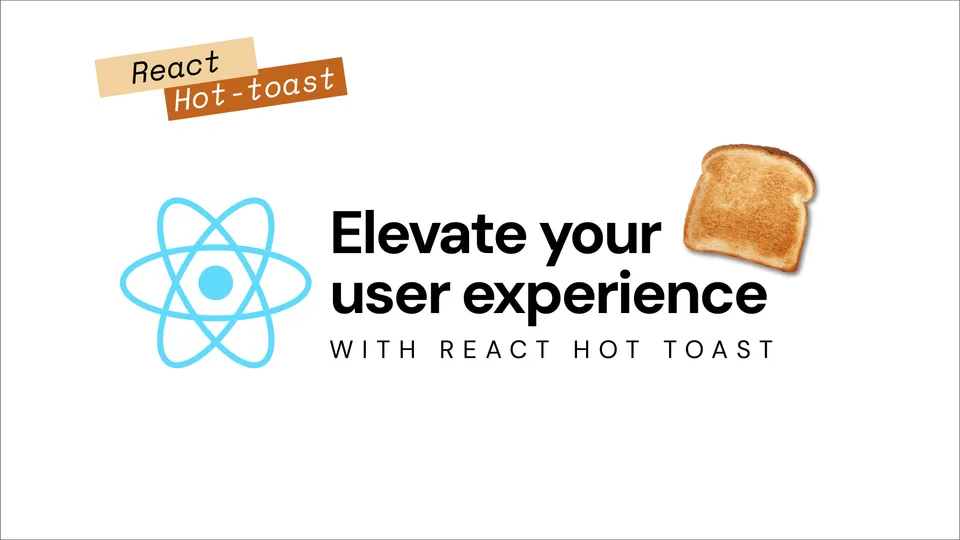
Mastering the Art of React Hot Toast: A Delectable Experience for Web Developers π₯
Share it on
Introduction to React Hot Toast π
Are you a web developer looking to add some sizzle to your React projects? Look no further than React Hot Toast! This powerful and versatile library allows you to easily implement stylish and interactive toast notifications in your applications. Whether you want to display success messages, errors, or simply provide feedback to your users, React Hot Toast has got you covered. With its intuitive API and extensive customization options, youβll be able to create beautiful and engaging toast messages in no time. From customizing the appearance and position of your toasts to adding animation and interactivity, React Hot Toast gives you complete control over the user experience. So why settle for plain and boring notifications when you can elevate your web applications to a whole new level with React Hot Toast? Get ready to master the art of React Hot Toast and delight your users with a delectable experience.
Why Use React Hot Toast? π€
- Simplicity: Easy to integrate and use.
- Customizability: Highly customizable with various options for appearance and behavior.
- Performance: Lightweight and performant.
- Accessibility: Supports ARIA accessibility standards.
Installation π οΈ
First, install React Hot Toast using npm or yarn:
npm install react-hot-toast
or
yarn add react-hot-toast
Basic Usage π
To get started with React Hot Toast, you need to wrap your application with the Toaster
component and then use the toast
function to display notifications.
Step 1: Wrap Your Application
In your main application file (e.g., App.js
or index.js
), wrap your app with the Toaster
component:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
import { Toaster } from 'react-hot-toast';
ReactDOM.render(
<React.StrictMode>
<App />
<Toaster />
</React.StrictMode>,
document.getElementById('root')
);
Step 2: Show Toasts
Use the toast
function to display notifications. You can call this function from anywhere in your component tree.
import React from 'react';
import toast from 'react-hot-toast';
function App() {
const notify = () => toast('Hello, world! π');
return (
<div>
<button onClick={notify}>Show Toast</button>
</div>
);
}
export default App;
Customization π¨
React Hot Toast allows you to customize the appearance and behavior of toasts.
Custom Styles
You can customize the styling of the toasts by passing a style object to the toast
function.
toast('This is a custom toast! β¨', {
style: {
border: '1px solid #713200',
padding: '16px',
color: '#713200',
},
});
Positioning
You can control the position of the toasts using the position
option. The available positions are top-left
, top-center
, top-right
, bottom-left
, bottom-center
, and bottom-right
.
toast('This toast is at the top-right! π', {
position: 'top-right',
});
Toast Types
React Hot Toast supports different types of toasts for different scenarios: success, error, loading, etc.
toast.success('Operation successful! π');
toast.error('Something went wrong! β');
toast.loading('Loading... β³');
Duration
Control how long the toast is displayed with the duration
option (in milliseconds).
toast('This toast will disappear in 1 second!', {
duration: 1000,
});
Real-Life Uses π
Form Submission Feedback
Provide instant feedback to users after form submission.
import React from 'react';
import toast from 'react-hot-toast';
function Form() {
const handleSubmit = (e) => {
e.preventDefault();
toast.success('Form submitted successfully! π');
};
return (
<form onSubmit={handleSubmit}>
<button type="submit">Submit</button>
</form>
);
}
export default Form;
Error Handling
Display error messages when something goes wrong.
import React from 'react';
import toast from 'react-hot-toast';
function FetchData() {
const fetchData = async () => {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
toast.success('Data fetched successfully! π¦');
} catch (error) {
toast.error(`Error: ${error.message} β`);
}
};
return (
<div>
<button onClick={fetchData}>Fetch Data</button>
</div>
);
}
export default FetchData;
Real-Time Updates
Notify users of real-time updates or events.
import React, { useEffect } from 'react';
import toast from 'react-hot-toast';
function RealTimeUpdates() {
useEffect(() => {
const interval = setInterval(() => {
toast('Real-time update! π');
}, 5000);
return () => clearInterval(interval);
}, []);
return (
<div>
<h1>Check your toasts for updates! π</h1>
</div>
);
}
export default RealTimeUpdates;
Conclusion π
React Hot Toast is a versatile and easy-to-use library for adding toast notifications to your React applications. Whether you need to notify users of successful operations, errors, or real-time updates, React Hot Toast has you covered. Its simplicity and customizability make it a great choice for enhancing user experience.
Give React Hot Toast a try in your next project and see how it can improve user interaction and feedback!