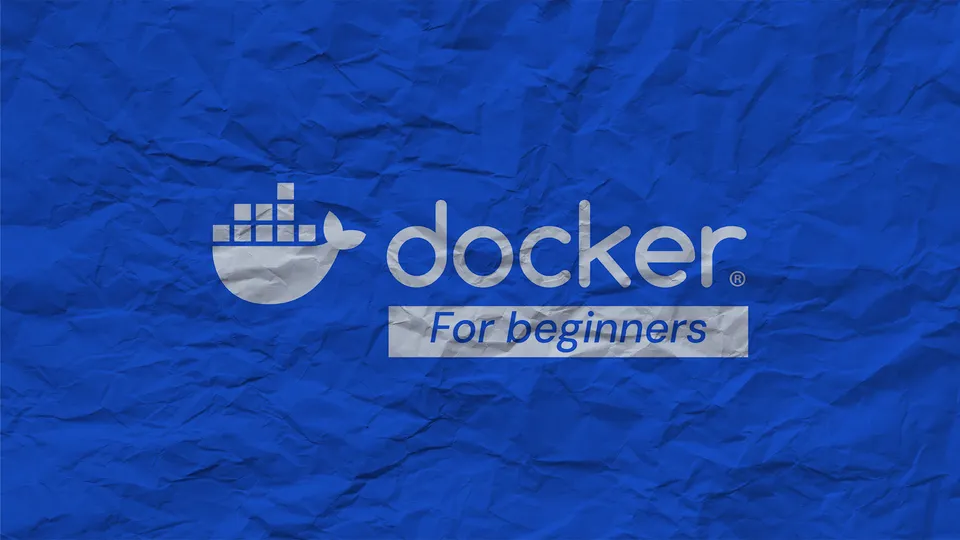
Docker is an open-source platform that automates the deployment, scaling, and management of applications using containerization. Containers are lightweight, stand-alone, executable packages that include everything needed to run a piece of software, including the code, runtime, libraries, and system tools.
Real-Life Scenario in Development Field
Scenario: Developing a Web Application
Development Without Docker:
-
Local Environment Setup:
- Developers set up their local environment manually, installing necessary software like Node.js, databases (e.g., MongoDB, PostgreSQL), and any other dependencies.
- Differences in operating systems and configurations can lead to “it works on my machine” problems, where the application runs on one developer’s machine but not on another’s.
-
Integration Issues:
- Integrating with different versions of software can cause compatibility issues.
- Ensuring that all team members are using the same versions of dependencies can be challenging.
-
Deployment Challenges:
- Moving from development to production often involves reconfiguring the application to fit the production environment.
- The process is error-prone and time-consuming.
Development With Docker:
-
Consistent Development Environment:
- Developers create a
Dockerfile
that specifies the environment configuration, including the base image (e.g.,node:14
), application code, and any dependencies. - A
docker-compose.yml
file can be used to define and run multi-container Docker applications, specifying services (e.g., web, database) and their configurations.
- Developers create a
-
Streamlined Integration:
- All team members use the same Docker images, ensuring consistent environments across all development machines.
- Developers can quickly spin up the entire application stack using Docker Compose, reducing setup time and avoiding compatibility issues.
-
Simplified Deployment:
- The same Docker images used in development can be deployed to production, ensuring consistency between environments.
- Docker simplifies scaling and managing the application in production using orchestration tools like Kubernetes.
Example Workflow with Docker:
-
Creating the Dockerfile:
# Use an official Node.js runtime as a parent image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy application files to the container COPY . . # Install application dependencies RUN npm install # Expose the application port EXPOSE 3000 # Define the command to run the application CMD ["npm", "start"]
-
Creating the Docker Compose File:
version: '3' services: web: build: . ports: - "3000:3000" db: image: mongo ports: - "27017:27017"
-
Running the Application:
-
To build and run the application, developers use the following command:
docker-compose up
-
This command creates and starts the containers for the web application and the MongoDB database.
-
Benefits:
- Consistency: Ensures the same environment is used across development, testing, and production.
- Isolation: Containers isolate applications from each other and the host system.
- Portability: Docker containers can run on any machine that has Docker installed, making it easy to move applications between environments.
- Efficiency: Containers share the host OS kernel, making them more lightweight and efficient than traditional virtual machines.
In summary, Docker simplifies the development and deployment process by providing a consistent, portable, and isolated environment for applications. This leads to faster development cycles, easier collaboration, and more reliable deployments.
How it works
Here’s an overview diagram for beginners to understand Docker and its components:
+-----------------------------+
| Docker Host |
| (Your Machine / Server) |
| |
| +----------------------+ |
| | Docker Daemon | |
| | (dockerd) | |
| +----------+-----------+ |
| | |
| | |
| +----------v-----------+ |
| | Docker CLI (Client)| |
| | (docker) | |
| +----------+-----------+ |
| | |
+-------------|---------------+
|
+-------------v---------------+
| Docker Architecture |
| |
| +----------------------+ |
| | Docker Images | |
| | (Stored Templates) | |
| +----------------------+ |
| |
| +----------------------+ |
| | Docker Containers | |
| | (Running Instances) | |
| +----------------------+ |
| |
+-----------------------------+
Components Explained:
-
Docker Host:
- The physical or virtual machine where Docker is installed.
- It runs the Docker Daemon and Docker CLI.
-
Docker Daemon (dockerd):
- The background service running on the Docker Host.
- Manages Docker objects (images, containers, networks, volumes).
-
Docker CLI (docker):
- The command-line interface for interacting with the Docker Daemon.
- Allows users to run Docker commands to manage images, containers, networks, and volumes.
-
Docker Images:
- Read-only templates used to create Docker containers.
- Can be pulled from Docker Hub or custom-built.
- Contains the application code, runtime, libraries, and dependencies.
-
Docker Containers:
- Running instances of Docker images.
- Lightweight and portable encapsulations of the application and its environment.
- Each container is isolated from others and the host system.
Workflow Example:
-
Creating a Docker Image:
- Write a
Dockerfile
that defines the image configuration. - Build the image using the command:
docker build -t myapp .
- Write a
-
Running a Docker Container:
- Run the container from the image using the command:
docker run -d -p 8080:80 myapp
- This command starts the container in detached mode and maps port 8080 on the host to port 80 on the container.
- Run the container from the image using the command:
-
Managing Containers:
- List running containers:
docker ps
- Stop a container:
docker stop <container_id>
- Remove a container:
docker rm <container_id>
- List running containers:
This diagram and explanation provide a high-level overview of Docker’s architecture and components for beginners.
How to setup docker for first time
Setting up Docker involves installing Docker Desktop (for Windows and macOS) or Docker Engine (for Linux) on your system. Here are the steps for each platform:
Windows and macOS:
-
Download Docker Desktop:
- Visit the Docker Desktop download page.
- Download the installer for your operating system (Windows or macOS).
-
Install Docker Desktop:
- Run the downloaded installer.
- Follow the installation instructions. On Windows, you may need to enable the WSL 2 feature if it’s not already enabled.
- After the installation, you might need to restart your computer.
-
Start Docker Desktop:
- Launch Docker Desktop from the Start menu (Windows) or Applications folder (macOS).
- Docker will start in the background, and you will see the Docker icon in the system tray (Windows) or menu bar (macOS).
-
Verify Installation:
-
Open a terminal or command prompt.
-
Run the following command to check if Docker is installed and running:
docker --version
-
You should see the Docker version output.
-
Linux:
-
Set Up the Repository:
-
Open a terminal.
-
Update your package index and install required packages:
sudo apt-get update sudo apt-get install \ ca-certificates \ curl \ gnupg \ lsb-release
-
Add Docker’s official GPG key:
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo gpg --dearmor -o /usr/share/keyrings/docker-archive-keyring.gpg
-
Set up the stable repository:
echo \ "deb [arch=$(dpkg --print-architecture) signed-by=/usr/share/keyrings/docker-archive-keyring.gpg] https://download.docker.com/linux/ubuntu \ $(lsb_release -cs) stable" | sudo tee /etc/apt/sources.list.d/docker.list > /dev/null
-
-
Install Docker Engine:
-
Update the package index:
sudo apt-get update
-
Install the latest version of Docker Engine and containerd:
sudo apt-get install docker-ce docker-ce-cli containerd.io
-
-
Verify Installation:
-
Run the following command to check if Docker is installed and running:
sudo docker --version
-
You should see the Docker version output.
-
-
Manage Docker as a Non-Root User (Optional):
-
To run Docker commands without
sudo
, add your user to thedocker
group:sudo usermod -aG docker $USER
-
Log out and log back in so that your group membership is re-evaluated.
-
Verify that you can run Docker commands without
sudo
:docker run hello-world
-
Post-Installation Steps:
-
Run a Test Container:
-
Run the
hello-world
container to verify that Docker is installed correctly:docker run hello-world
-
You should see a message that confirms Docker is working.
-
-
Pull and Run Images:
-
Pull an image from Docker Hub:
docker pull nginx
-
Run the pulled image:
docker run -d -p 80:80 nginx
-
This command runs an Nginx server in a container, mapping port 80 of the host to port 80 of the container.
-
-
Explore Docker Commands:
-
List running containers:
docker ps
-
List all containers (including stopped ones):
docker ps -a
-
Stop a running container:
docker stop <container_id>
-
Remove a container:
docker rm <container_id>
-
Remove an image:
docker rmi <image_id>
-
These steps will help you set up Docker on your system and get started with containerization.