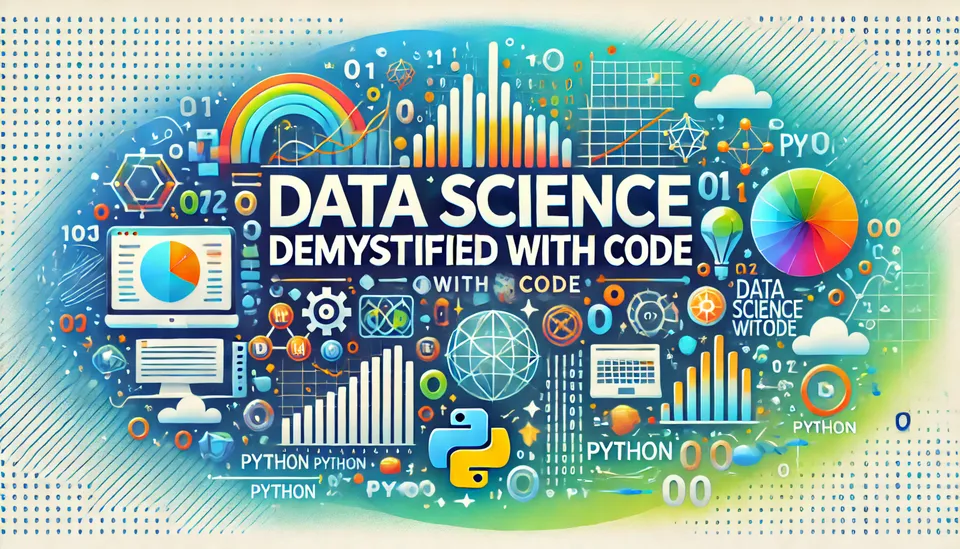
Data Science is everywhere! From predicting trends to making data-driven decisions, itโs a field that is revolutionizing industries and careers. But where do you start? How do you break down the complexities? Letโs demystify Data Science together, step by step, using Python with hands-on code examples. ๐๐ป
In this blog, weโll dive into the exciting world of Data Science and show you how to collect, clean, explore, and model data to uncover valuable insights. Letโs get started! ๐
1. ๐ What is Data Science?
Data Science is a blend of statistics, computer science, and machine learning that helps us extract meaningful insights from raw data. Think of it as a treasure hunt ๐ดโโ ๏ธ where the treasure is the knowledge you gain from analyzing data!
The typical steps in a Data Science project are:
- Data Collection: Gathering the data ๐ฅ
- Data Cleaning: Making the data ready for analysis ๐งน
- Exploratory Data Analysis (EDA): Visualizing and understanding the data ๐
- Modeling: Building machine learning models to make predictions ๐ค
- Deployment: Putting models to work in real applications ๐
Letโs break down each step with some practical examples.
2. ๐ Data Collection
Before we analyze, we need data! One popular dataset is the Iris dataset, which contains information about flowers ๐ธ. Weโll use this to start our Data Science journey.
from sklearn.datasets import load_iris
import pandas as pd
# Load the Iris dataset
iris = load_iris()
# Convert to DataFrame for easier manipulation
df = pd.DataFrame(iris.data, columns=iris.feature_names)
df['species'] = iris.target
# Display the first few rows of the dataset
print(df.head())
Sample Output:
sepal length (cm) sepal width (cm) petal length (cm) petal width (cm) species
0 5.1 3.5 1.4 0.2 0
1 4.9 3.0 1.4 0.2 0
2 4.7 3.2 1.3 0.2 0
3 4.6 3.1 1.5 0.2 0
4 5.0 3.6 1.4 0.2 0
Now we have our data ready! Letโs move on to cleaning it up. ๐งฝ
3. ๐งน Data Cleaning
Real-world data can be messy. ๐ฌ Itโs our job as Data Scientists to clean and preprocess it before diving into analysis. Weโll scale the features here to make sure all data is on the same scale. This helps the algorithms work better.
from sklearn.preprocessing import StandardScaler
# Scale the features
scaler = StandardScaler()
scaled_features = scaler.fit_transform(df.drop('species', axis=1))
# Convert back to DataFrame for easier viewing
scaled_df = pd.DataFrame(scaled_features, columns=df.columns[:-1])
print(scaled_df.head())
Now the data is clean and ready for exploration! ๐จ
4. ๐จ Exploratory Data Analysis (EDA)
Exploring the data through visualizations helps us understand its patterns and relationships. Letโs visualize the relationships between the features using Seaborn and Matplotlib.
import matplotlib.pyplot as plt
import seaborn as sns
# Visualizing the distribution of features
sns.pairplot(df, hue='species', palette='viridis')
plt.show()
This generates a scatter plot matrix, showing the relationships between different features of the Iris dataset. ๐ผ๐ซ
5. ๐ค Building a Machine Learning Model
Now comes the exciting part โ building a machine learning model! For this task, weโll use Logistic Regression to predict the species of a flower ๐ธ based on its features. Itโs a simple yet powerful algorithm.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, confusion_matrix
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(scaled_df, df['species'], test_size=0.2, random_state=42)
# Build the logistic regression model
model = LogisticRegression()
model.fit(X_train, y_train)
# Make predictions
y_pred = model.predict(X_test)
# Evaluate the model
print("Accuracy:", accuracy_score(y_test, y_pred))
print("Confusion Matrix:\n", confusion_matrix(y_test, y_pred))
Sample Output:
Accuracy: 1.0
Confusion Matrix:
[[10 0 0]
[ 0 9 0]
[ 0 0 11]]
Wow! Our model has 100% accuracy on the test data! ๐
6. ๐ Conclusion
Data Science is like unlocking a hidden world of insights that can change the way we think and act. In this blog, weโve covered the basics: from collecting data to building a machine learning model. Weโve seen how Python is the go-to tool for Data Science, and with libraries like pandas, seaborn, and scikit-learn, it becomes a breeze to work with data. ๐
Key Takeaways:
- Data Science is an exciting field that empowers us to uncover patterns and make informed decisions.
- Python and its libraries (like scikit-learn, pandas, matplotlib) make it easy to manipulate, explore, and model data.
- Machine learning can help us predict outcomes, and with just a few lines of code, we can build powerful models!
The journey doesnโt end here. As you dive deeper into Data Science, youโll explore more advanced topics like Deep Learning, Natural Language Processing (NLP), and AI. ๐
Happy coding, and welcome to the world of Data Science! ๐