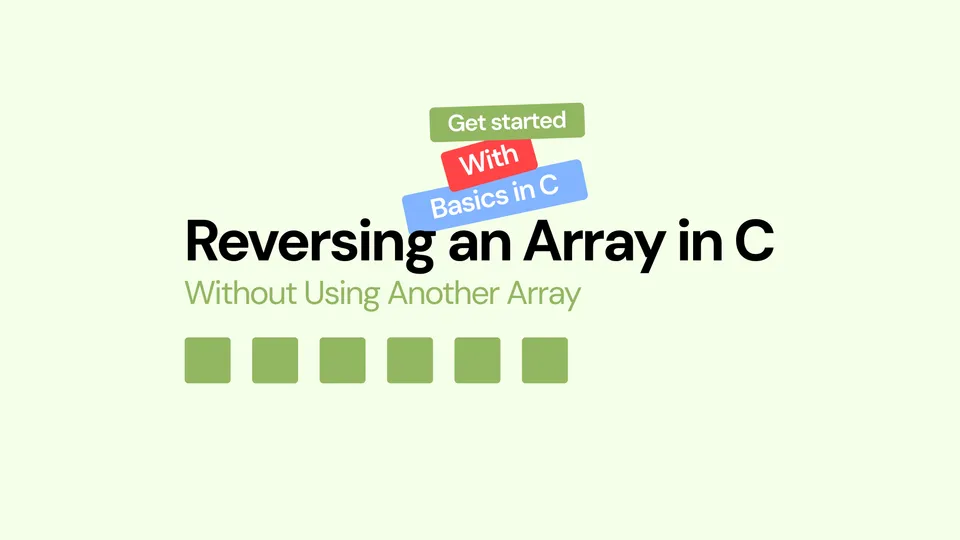
π§βπ» Basics in C: Reversing an Array in C Without Using Another Array
Share it on
π Description:
In this blog post, weβll explore how to reverse an array in C without the need for an additional array. This technique is efficient and demonstrates the power of in-place algorithms, where the original data structure is modified directly without requiring extra memory. Weβll break down the code step by step and provide examples to illustrate how it works.
π Understanding the Code
The program begins by asking the user to input the size of the array and then the elements of the array. It then displays the array as entered. The key part of the program is the logic that reverses the array by swapping elements from the ends towards the center, eliminating the need for another array. Finally, it displays the reversed array.
Code:
#include<stdio.h>
int main(){
int n,i,t;
printf("Enter size of the array : ");
scanf("%d", &n);
int a[n];
printf("Enter values into the array : \n");
for(i=0;i<n;i++){
printf("a[%d] : ", i);
scanf("%d", &a[i]);
}
printf("The array is given below : \n");
for(i=0;i<n;i++)
printf("%d ", a[i]);
for(i=0;i<n/2;i++){
t=a[n-1-i];
a[n-1-i]=a[i];
a[i]=t;
}
printf("\nAfter reversing, the array is given below : \n");
for(i=0;i;n;i++)
printf("%d ", a[i]);
return 0;
}
π Example 1: Reversing an Array of 5 Elements
Letβs consider an array of 5 elements: [1, 2, 3, 4, 5]
.
-
Input:
Enter size of the array : 5 Enter values into the array : a[0] : 1 a[1] : 2 a[2] : 3 a[3] : 4 a[4] : 5
-
Output:
The array is given below : 1 2 3 4 5 After reversing, the array is given below : 5 4 3 2 1
π Explanation:
Here, the program reverses the array by swapping the first element with the last, the second with the second last, and so on. The middle element remains unchanged.
π Example 2: Reversing an Array of 6 Elements
Now, letβs reverse an array of 6 elements: [10, 20, 30, 40, 50, 60]
.
-
Input:
Enter size of the array : 6 Enter values into the array : a[0] : 10 a[1] : 20 a[2] : 30 a[3] : 40 a[4] : 50 a[5] : 60
-
Output:
The array is given below : 10 20 30 40 50 60 After reversing, the array is given below : 60 50 40 30 20 10
π Explanation:
In this case, the program swaps elements starting from the outermost pairs and works its way inward. As a result, the array is perfectly reversed.
π Conclusion
Reversing an array without using an additional array is a simple yet effective technique that minimizes memory usage. By understanding and applying this method, you can enhance the performance of your programs, especially when dealing with large datasets. Happy coding! π